-->
Home / ASP.NET Forums / Data Access / SQL Server. How to insert product in shopping cart in a new row if exists but the color and size is different? [Answered] RSS. (36), @ProductId int, @Attributes nvarchar(1000)) AS -- update quantity and if it is same cart info Update ShoppingCart SET Quantity = Quantity + 1 Where. Continuing the concept further this article will illustrate how to use store your shopping cart in a database. ASP.NET Core 2.2 and Angular 7: Instructor-led. My college professor wants me to write a ASP.NET program using C# that has a shopping cart that does not connect to a database. He says we can put some static data in using C#. He was a list of possible items to show up.then the customer can add the item. In the shopping cart the customer can choose the quantity then update the cart. User can change item count and click update button and item count will change in DataTable. Sir i needed simple shopping cart done asp.net. Shajahan saju. Home / ASP.NET Forums /.NET Languages / Visual Basic.NET / Shopping Cart Can't Update Quantity Shopping Cart Can't Update Quantity [Answered] RSS 2 replies. ASP Repeater - Shopping cart quantity problem. When the user will clicks on the ADD TO CART button. Exploring ASP.NET Core: Fundamentals. To manage shopping cart access, you will assign users a unique ID using a globally unique identifier (GUID) when the user accesses the shopping cart for the first time. You'll store this ID using the ASP.NET Session state.
by Jon Galloway
The MVC Music Store is a tutorial application that introduces and explains step-by-step how to use ASP.NET MVC and Visual Studio for web development.
The MVC Music Store is a lightweight sample store implementation which sells music albums online, and implements basic site administration, user sign-in, and shopping cart functionality.
This tutorial series details all of the steps taken to build the ASP.NET MVC Music Store sample application. Part 8 covers Shopping Cart with Ajax Updates.
We'll allow users to place albums in their cart without registering, but they'll need to register as guests to complete checkout. The shopping and checkout process will be separated into two controllers: a ShoppingCart Controller which allows anonymously adding items to a cart, and a Checkout Controller which handles the checkout process. We'll start with the Shopping Cart in this section, then build the Checkout process in the following section.
Adding the Cart, Order, and OrderDetail model classes
Our Shopping Cart and Checkout processes will make use of some new classes. Right-click the Models folder and add a Cart class (Cart.cs) with the following code.
This class is pretty similar to others we've used so far, with the exception of the [Key] attribute for the RecordId property. Our Cart items will have a string identifier named CartID to allow anonymous shopping, but the table includes an integer primary key named RecordId. By convention, Entity Framework Code-First expects that the primary key for a table named Cart will be either CartId or ID, but we can easily override that via annotations or code if we want. This is an example of how we can use the simple conventions in Entity Framework Code-First when they suit us, but we're not constrained by them when they don't.
Next, add an Order class (Order.cs) with the following code.
This class tracks summary and delivery information for an order. It won't compile yet, because it has an OrderDetails navigation property which depends on a class we haven't created yet. Let's fix that now by adding a class named OrderDetail.cs, adding the following code.
We'll make one last update to our MusicStoreEntities class to include DbSets which expose those new Model classes, also including a DbSet<Artist>. The updated MusicStoreEntities class appears as below.
Managing the Shopping Cart business logic
Next, we'll create the ShoppingCart class in the Models folder. The ShoppingCart model handles data access to the Cart table. Additionally, it will handle the business logic to for adding and removing items from the shopping cart.
Since we don't want to require users to sign up for an account just to add items to their shopping cart, we will assign users a temporary unique identifier (using a GUID, or globally unique identifier) when they access the shopping cart. We'll store this ID using the ASP.NET Session class.
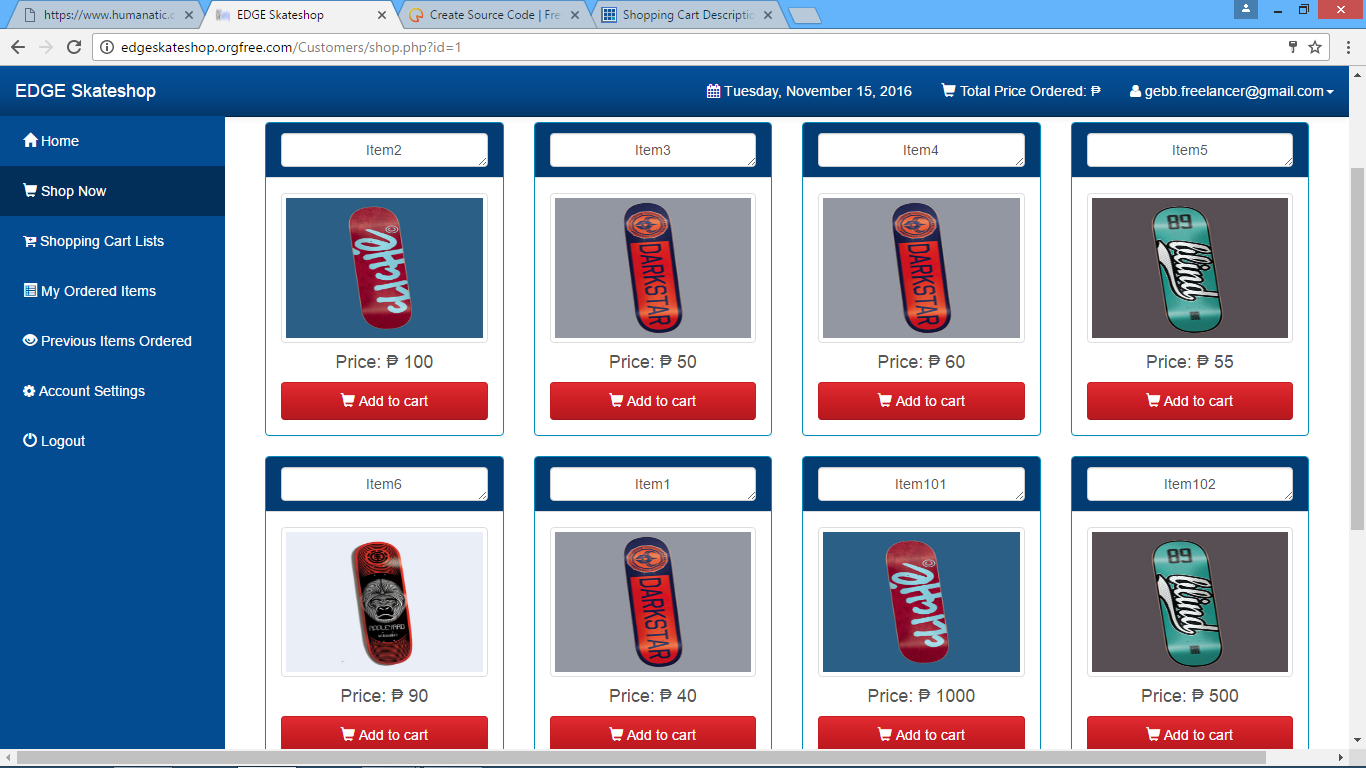
Note: The ASP.NET Session is a convenient place to store user-specific information which will expire after they leave the site. While misuse of session state can have performance implications on larger sites, our light use will work well for demonstration purposes.
The ShoppingCart class exposes the following methods:
AddToCart takes an Album as a parameter and adds it to the user's cart. Since the Cart table tracks quantity for each album, it includes logic to create a new row if needed or just increment the quantity if the user has already ordered one copy of the album.
RemoveFromCart takes an Album ID and removes it from the user's cart. If the user only had one copy of the album in their cart, the row is removed.
EmptyCart removes all items from a user's shopping cart.
GetCartItems retrieves a list of CartItems for display or processing.
GetCount retrieves a the total number of albums a user has in their shopping cart.
GetTotal calculates the total cost of all items in the cart.
CreateOrder converts the shopping cart to an order during the checkout phase.
GetCart is a static method which allows our controllers to obtain a cart object. It uses the GetCartId method to handle reading the CartId from the user's session. The GetCartId method requires the HttpContextBase so that it can read the user's CartId from user's session.

Here's the complete ShoppingCart class:
ViewModels
Our Shopping Cart Controller will need to communicate some complex information to its views which doesn't map cleanly to our Model objects. We don't want to modify our Models to suit our views; Model classes should represent our domain, not the user interface. One solution would be to pass the information to our Views using the ViewBag class, as we did with the Store Manager dropdown information, but passing a lot of information via ViewBag gets hard to manage.
A solution to this is to use the ViewModel pattern. When using this pattern we create strongly-typed classes that are optimized for our specific view scenarios, and which expose properties for the dynamic values/content needed by our view templates. Our controller classes can then populate and pass these view-optimized classes to our view template to use. This enables type-safety, compile-time checking, and editor IntelliSense within view templates.
We'll create two View Models for use in our Shopping Cart controller: the ShoppingCartViewModel will hold the contents of the user's shopping cart, and the ShoppingCartRemoveViewModel will be used to display confirmation information when a user removes something from their cart.
Let's create a new ViewModels folder in the root of our project to keep things organized. Right-click the project, select Add / New Folder.
Name the folder ViewModels.
Next, add the ShoppingCartViewModel class in the ViewModels folder. It has two properties: a list of Cart items, and a decimal value to hold the total price for all items in the cart.
Now add the ShoppingCartRemoveViewModel to the ViewModels folder, with the following four properties.
The Shopping Cart Controller
The Shopping Cart controller has three main purposes: adding items to a cart, removing items from the cart, and viewing items in the cart. It will make use of the three classes we just created: ShoppingCartViewModel, ShoppingCartRemoveViewModel, and ShoppingCart. As in the StoreController and StoreManagerController, we'll add a field to hold an instance of MusicStoreEntities.
Add a new Shopping Cart controller to the project using the Empty controller template.
Here's the complete ShoppingCart Controller. The Index and Add Controller actions should look very familiar. The Remove and CartSummary controller actions handle two special cases, which we'll discuss in the following section.
Ajax Updates with jQuery
Update Quantity Shopping Cart Asp Net Users
We'll next create a Shopping Cart Index page that is strongly typed to the ShoppingCartViewModel and uses the List View template using the same method as before.
However, instead of using an Html.ActionLink to remove items from the cart, we'll use jQuery to 'wire up' the click event for all links in this view which have the HTML class RemoveLink. Rather than posting the form, this click event handler will just make an AJAX callback to our RemoveFromCart controller action. The RemoveFromCart returns a JSON serialized result, which our jQuery callback then parses and performs four quick updates to the page using jQuery:
- Removes the deleted album from the list
- Updates the cart count in the header
- Displays an update message to the user
- Updates the cart total price
Since the remove scenario is being handled by an Ajax callback within the Index view, we don't need an additional view for RemoveFromCart action. Here is the complete code for the /ShoppingCart/Index view:
In order to test this out, we need to be able to add items to our shopping cart. We'll update our Store Details view to include an 'Add to cart' button. While we're at it, we can include some of the Album additional information which we've added since we last updated this view: Genre, Artist, Price, and Album Art. The updated Store Details view code appears as shown below.
Now we can click through the store and test adding and removing Albums to and from our shopping cart. Run the application and browse to the Store Index.
Next, click on a Genre to view a list of albums.
Clicking on an Album title now shows our updated Album Details view, including the 'Add to cart' button.
Clicking the 'Add to cart' button shows our Shopping Cart Index view with the shopping cart summary list.
Asp Shopping Cart Software
After loading up your shopping cart, you can click on the Remove from cart link to see the Ajax update to your shopping cart.
We've built out a working shopping cart which allows unregistered users to add items to their cart. In the following section, we'll allow them to register and complete the checkout process.
Shopping carts are very important and can many times be the most intimidating part of building an e-commerce site. This tutorial will show how easy it can be to implement a shopping cart using ASP.NET. Additionally, several basic explanations will be provided to help beginning ASP.NET programmers understand this wonderful framework.
Quick Overview of ASP.NET
Since ASP.NET hasn't been covered too much on NETTUTS, I thought it would be good to include a brief overview of some of the things that distinguish it from other languages.
- Code is compiled. The first time an ASP.NET page is requested over the web, the code is compiled into one or more DLL files on the server. This gives you the ability to just copy code out to the server and it gives you the speed benefit of compiled code.
- ASP.NET is an object oriented framework. Every function, property and page is part of a class. For example, each web page is its own class that extends the Page class. The Page class has an event that is fired when the webpage is loaded called the 'Page Load Event'. You can write a function that subscribes to that event and is called on. The same principle applies to other events like the button click and 'drop-down' 'selected index changed' events.
- The logic is separate from the design and content. They interact with each other, but they are in separate places. Generally, this allows a designer to design without worrying about function and it allows the programmer to focus on function without looking at the design. You have the choice of putting them both in the same file or in different files. This is similar to model-view-controller model.

If you are new to ASP.NET (and you have Windows), you can try it out for free You can download Visual Studio Express by visiting the ASP.NET website. Also, when you create a website locally on your machine, you can run the website at any time and Visual Studio will quickly start a server on your computer and pull up your website in your default browser.
Step 1: Create the ShoppingCart Class
We need a place to store the items in the shopping cart as well as functions to manipulate the items. We'll create a ShoppingCart class for this. This class will also manage session storage.
First, we have to create the App_Code folder. To do this, go to the 'Website' menu, then 'Add ASP.NET Folder', and choose 'App_Code.' This is where we'll put all of our custom classes. These classes will automatically be accessible from the code in any of our pages (we don't need to reference it using something similar to 'include' or anything). Then we can add a class to that folder by right-clicking on the folder and choosing 'Add New Item.'
Quick Tip: Regions in ASP.NET are really nice to organize and group code together. The nicest thing about them is that you can open and close regions to minimize the amount of code that you are looking at or quickly find your way around a file.
Step 2: The CartItem & Product Classes
With a place to store our shopping cart items, we need to be able to store information about each item. We'll create a CartItem class that will do this. We'll also create a simple Product class that will simulate a way to grab data about the products we're selling.
The CartItem class:
The Product class:
Definition: A 'property' in ASP.NET is a variable in a class that has a setter, a getter, or both. This is similar to other languages, but in ASP.NET, the word property refers specifically to this. An example of this is the ProductId property in the CartItem class. It is not simply a variable in a class with a method to get or set it. It is declared in a special way with get{} and set{} blocks.
Let's Add Items to the Cart
After having our heads in the code for so long, it's time we do something visual. This page will simply be a way to add items to the cart. All we need is a few items with 'Add to Cart' links. Let's put this code in the Default.aspx page.
As you can see, the only thing happening here is that we have a few LinkButtons that have OnClick event handlers associated to them.
In the code-behind page, we have 4 event handlers. We have one for each LinkButton that just adds an item to the shopping cart and redirects the user to view their cart. We also have a Page_Load event handler which is created by the IDE by default that we didn't need to use.
Build the Shopping Cart Page
Finally, what we've been preparing for the whole time—the shopping cart! Let's just look at ViewCart.aspx first and I'll explain it after that.
The GridView control is a powerful control that can seem complicated at first. I won't discuss the style elements because they are self-explanatory. (There are some principles here that I'm not going to explain in depth. I am just going to try to get the main idea across). Let's break it down.
- Giving the GridView an ID will allow us to access the GridView from the code-behind using that ID.
- The GridView will automatically generate columns and column names from the data that we supply unless we specifically tell it not to.
- We can tell the GridView what to display in case we supply it with no data.
- We want to show the footer so that we can display the total price.
- It will be nice for us to have an array of ProductIds indexed by the row index when we are updating the quantity of a cart item in the code-behind. This will do that for us:
- We need events to respond to two events: RowDataBound and RowCommand. Basically, RowDataBound is fired when the GridView takes a row of our data and adds it to the table. We are only using this event to respond to the footer being bound so that we can customize what we want displayed there. RowCommand is fired when a link or a button is clicked from inside the GridView. In this case, it is the 'Remove' link.
Now let's talk about the columns. We define the columns here and the GridView will take every row in the data that we supply and map the data in that row to the column that it should display in. The simplest column is the BoundField. In our case, it is going to look for a 'Description' property in our CartItem object and display it in the first column. The header for that column will also display 'Description.'
We needed the quantity to display inside a textbox rather than just displaying as text, so we used a TemplateField. The TemplateField allows you to put whatever you want in that column. If you need some data from the row, you just insert <%# Eval('PropertyName') %>. The LinkButton that we put in our TemplateField has a CommandName and a CommandArgument, both of which will be passed to our GridView's RowCommand event handler.
The last thing worth mentioning here is that the last two BoundFields have a DataFormatString specified. This is just one of the many format strings that ASP.NET provides. This one formats the number as a currency. See the Microsoft documentation for other format strings.
Now we can look at the code-behind page. I have supplied lots of comments here to describe what is happening.
The End Result:
Now we have a nice working shopping cart!
You Also Might Like...
Oct 1st in Screencasts by Jeffrey Way
I’m happy to say that today, we are posting our very first article on ASP.NET. In this screencast, I’ll show you how to implement a simple search functionality into your personal website. We’ll go over many of the new features in ASP.NET 3.5, such as LINQ and many of the AJAX controls that ship with Visual Studio/Web Developer.
- Subscribe to the NETTUTS RSS Feed for more daily web development tuts and articles.